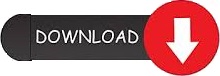
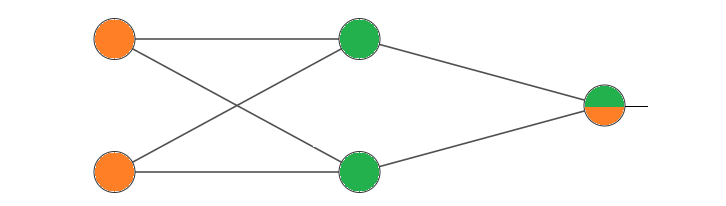
- Python merge two dictionaries how to#
- Python merge two dictionaries generator#
- Python merge two dictionaries update#
- Python merge two dictionaries code#
Naive Method to Combine two dictionary adding values for common keys Here we are iterating over the dictionarie s and adding the values for the same keys. To combine dicts in Python, use the dict.update () method.
Python merge two dictionaries how to#
(Self-contained so you can copy and paste yourself. Let’s see some of the methods on How to Combine two dictionaries by adding values for common keys in Python. I’m only going to do the performance analysis of the usages known to behave correctly.
Python merge two dictionaries update#
Update () method in python update () method is to update one of the dictionaries with the key and value of another dictionary.

Method 1- Using update () method Before understanding this let us understand how the update () method works in python.
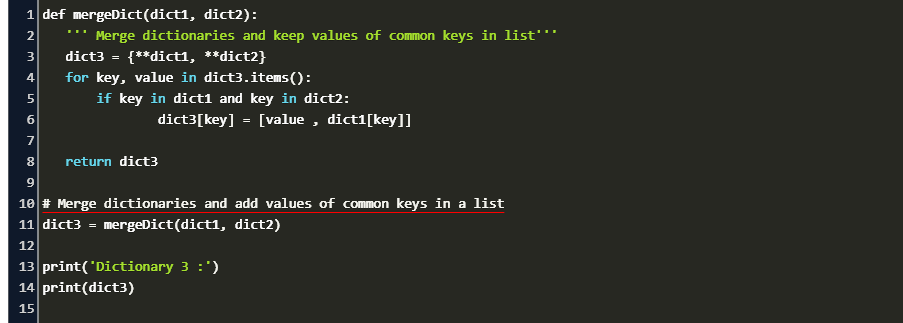
Z = dict(chain(x.items(), y.items())) # iteritems in Python 2 Performance Analysis Dict In Python Sort Dict in Python Add Key In Dict in Python Concatenate New Dict in Python Check Key Already Exitsts Dict in Python Loop in Dict in Python. In this article, we discuss how to merge two or more dictionaries in python. Itertools.chain will chain the iterators over the key-value pairs in the correct order: from itertools import chain
Python merge two dictionaries generator#
Or in python 2.6 (and perhaps as early as 2.4 when generator expressions were introduced): dict((k, v) for d in dicts for k, v in d.items()) # iteritems in Python 2 Say you have two dictionaries and you want to merge them into a new dict without altering the original dictionaries: x = # iteritems in Python 2.7
Python merge two dictionaries code#
Some of the methods need a few lines of code to merge while one can combine the dictionaries in a single expression. The dictionary has a built-in method dict.update() that merges the key-value pairs of the other dictionary to the current dictionary object. Z.update(y) # modifies z with y's keys and values & returns None return z Sample Solution-2: Create a new dict and loop over dicts, using dictionary.update() to add the key-value pairs from each one to the result. There is no built-in function to concatenate (merge) two dictionaries together in Python, but we can make some arrangements to do that. Summary: In this tutorial, we will learn different ways to merge two or more dictionaries in Python. Method 1: Using Counter Counter is a special subclass of dictionary which performs acts same as dictionary in most cases. Let’s see how to combine the values of two dictionaries having same key. list1 1, 2, 2, 5 list2 2, 5, 7, 9 res list (set (list1 + list2)) print (res) print (list1) print (list2) You can do also convert the list to a new set. Recall that dictionaries are mutable objects and that the statement merged dict1 simply assigns a variable that references dict1 rather than creating a new copy of the dictionary. Use set to remove duplicate without removing element from the original list. The problem with our function is that we inadvertently merge dict2 into dict1, rather than merging the two dictionaries into a new dictionary. Z = x.copy() # start with x's keys and values Combining dictionaries is very common task in operations of dictionary. Combining two lists and without duplicates and don’t remove duplicates in the original list. In Python 2, (or 3.4 or lower) write a function: def merge_two_dicts( x, y): In Python 3.9.0 or greater (released 17 October 2020): PEP-584, discussed here, was implemented and provides the simplest method: z = x | y # NOTE: 3.9+ ONLY The second list of dictionary is iterated over, and if the keys in this are not present in the previous variable, the specific keys from both the lists are equated.Answer #1: How can I merge two Python dictionaries in a single expression?įor dictionaries x and y, z becomes a shallowly merged dictionary with values from y replacing those from x. The list of dictionary is iterated over and the keys are accessed.
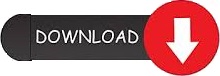